Introduction to Programming Concepts
1. Sending Keys/Mouse
Sending keys/mouse
Automating keyboard and mouse actions is like having a ghostwriter for your computer. Just as a ghostwriter pens words on behalf of someone else, automation scripts perform clicks and keystrokes as if an invisible hand were controlling the computer, executing tasks without the need for human intervention.
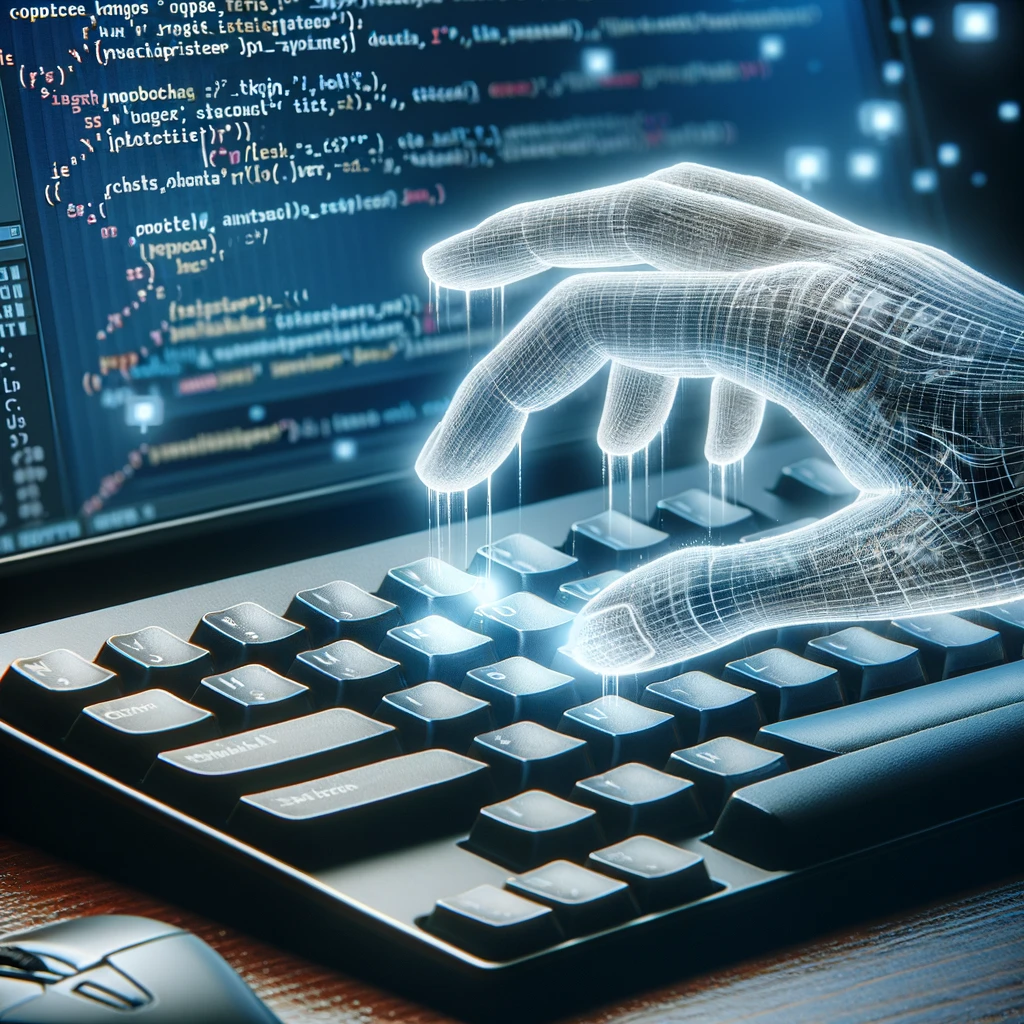
2. Hotkeys
Hotkeys
Hotkeys are the secret passages of software applications. Much like a hidden door in a bookshelf that leads to a secret room, pressing a combination of keys swiftly transports you to a specific feature or action within a program, bypassing the need to navigate through a maze of menus.
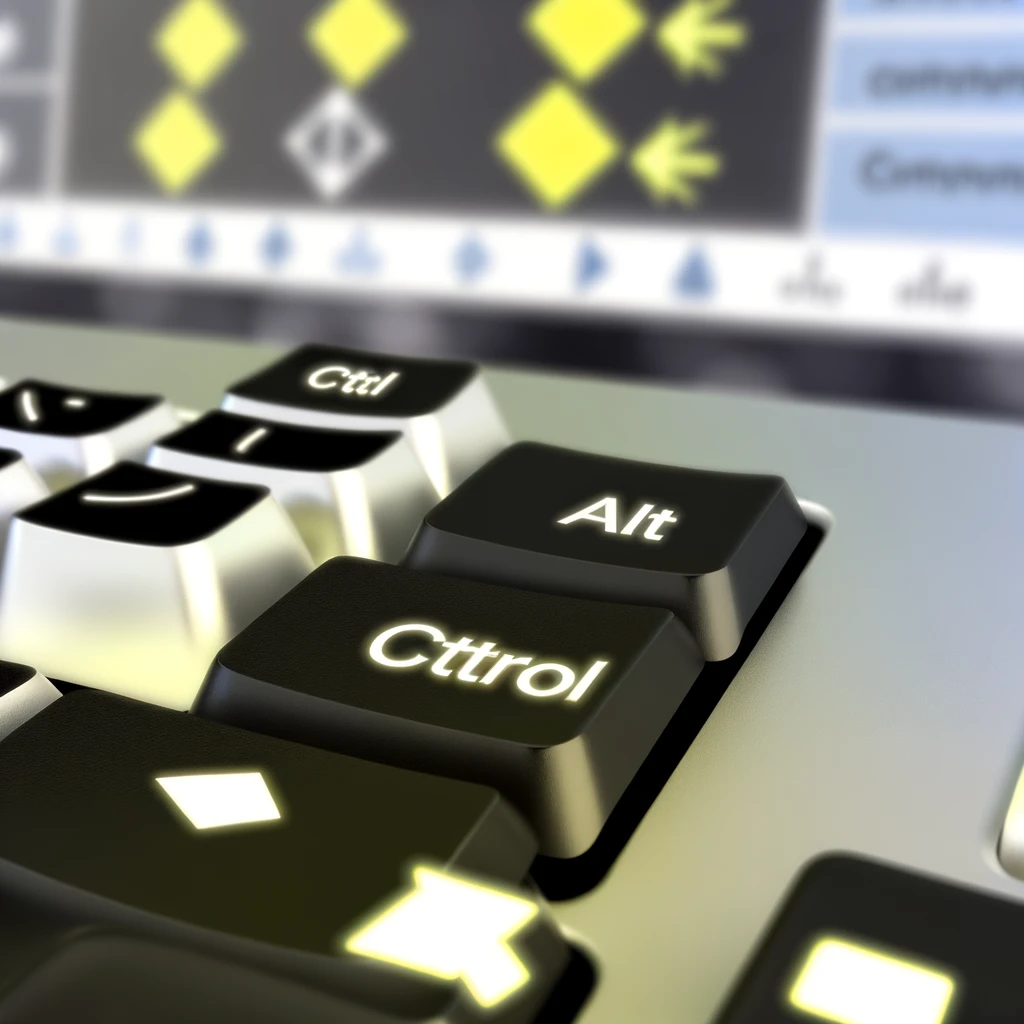
3. GUI (Graphical User Interface)
GUI
A Graphical User Interface (GUI) is like the dashboard of a car. Just as a car's dashboard provides buttons and displays to control the vehicle and see its status at a glance, a GUI offers graphical elements such as icons, buttons, and windows that allow users to interact with a software application easily and intuitively.
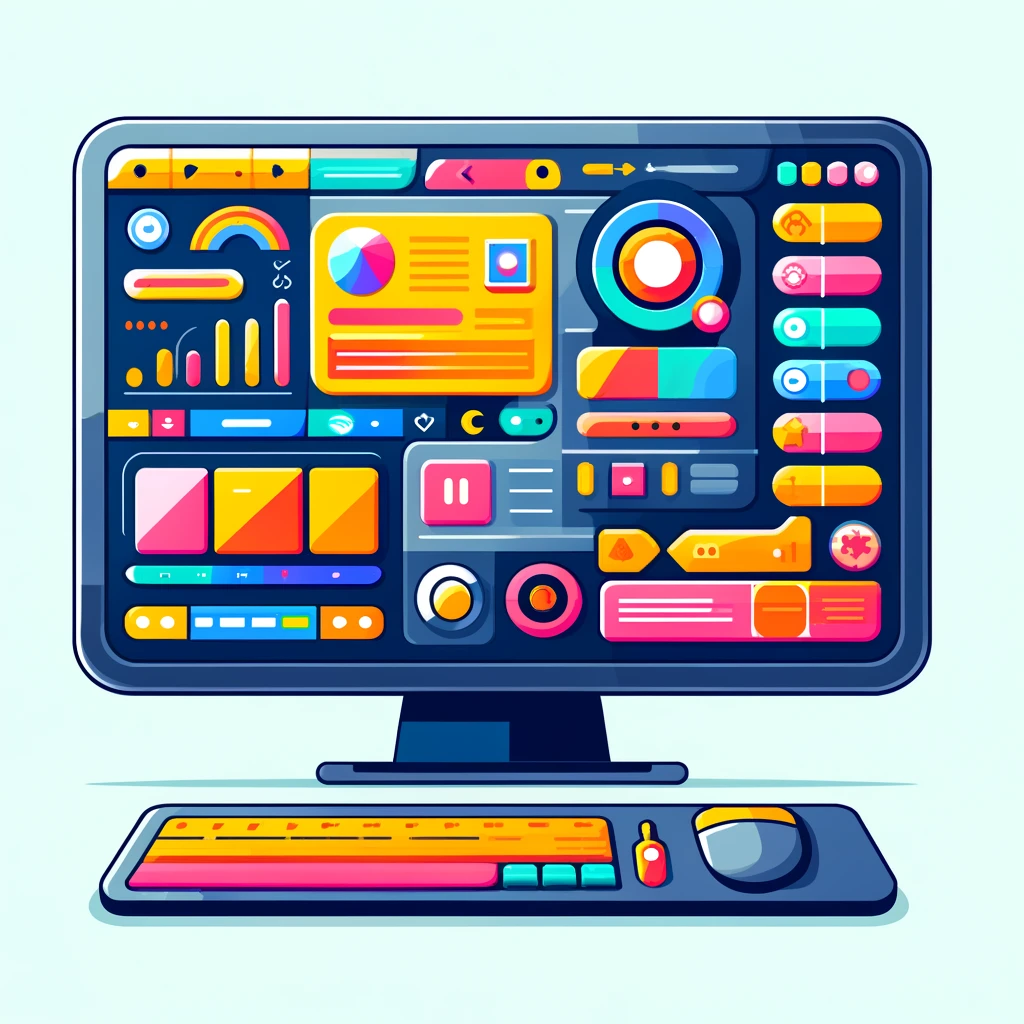
4. RegEx (Regular Expressions)
Regular Expressions (RegEx)
Regular Expressions (RegEx) are the Swiss Army knives of text processing. Just as a Swiss Army knife contains various tools to perform a wide range of tasks, RegEx allows you to wield a single, powerful tool to search, edit, and manipulate text in complex and versatile ways.
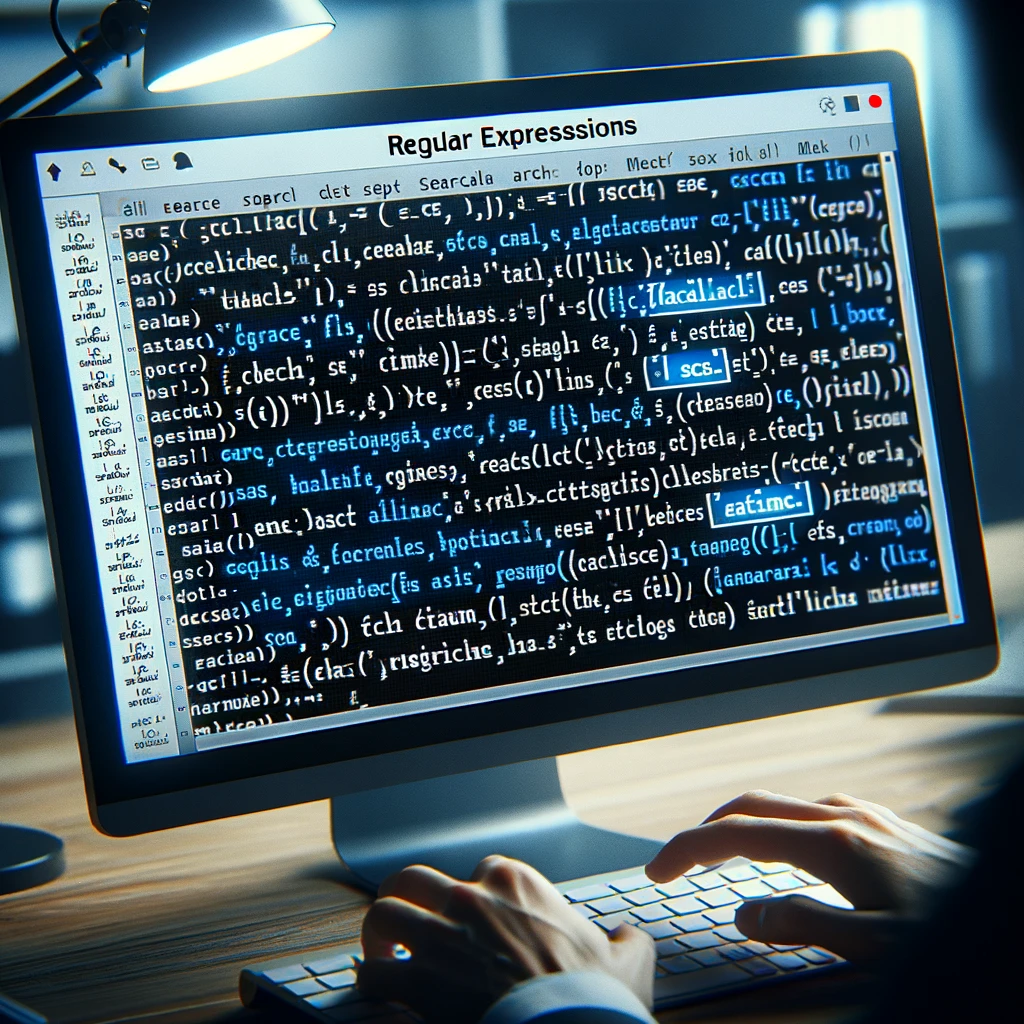
5. WinAPI (Windows API)
WinAPI
The Windows API (WinAPI) is like the backstage of a theater. While the audience (the users) sees the performance (the software application) on stage, the backstage (WinAPI) is where all the behind-the-scenes magic happens, enabling the show to go on by providing essential services and resources that the actors (applications) rely on to perform.
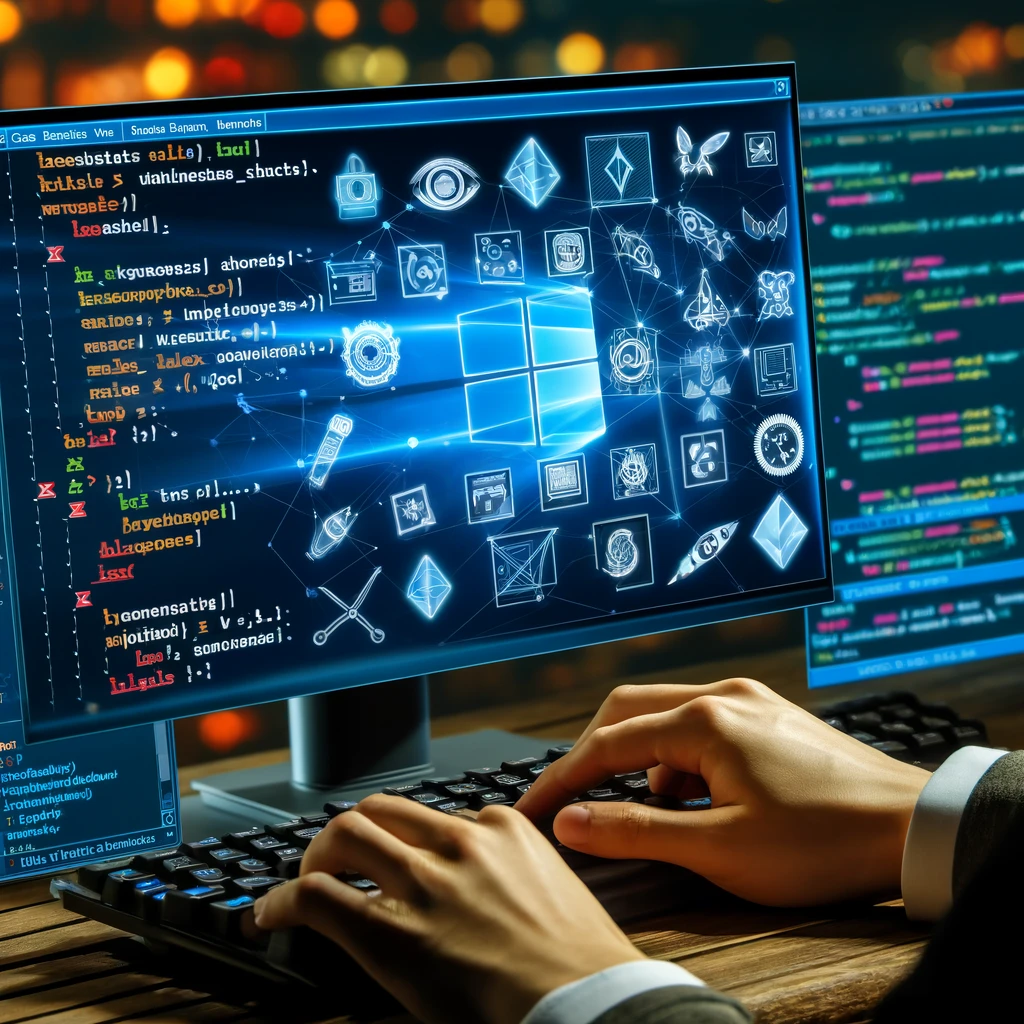
6. COM Objects (Component Object Model)
COM Objects
COM Objects are like the universal power adapters of the software world. Just as a power adapter allows devices from different countries with various plug shapes and electrical standards to work seamlessly with the power sockets of another country, COM Objects enable software components to communicate and work together, regardless of the languages or technologies they were developed in. They act as a bridge, ensuring that diverse software components can connect and function as a cohesive unit.
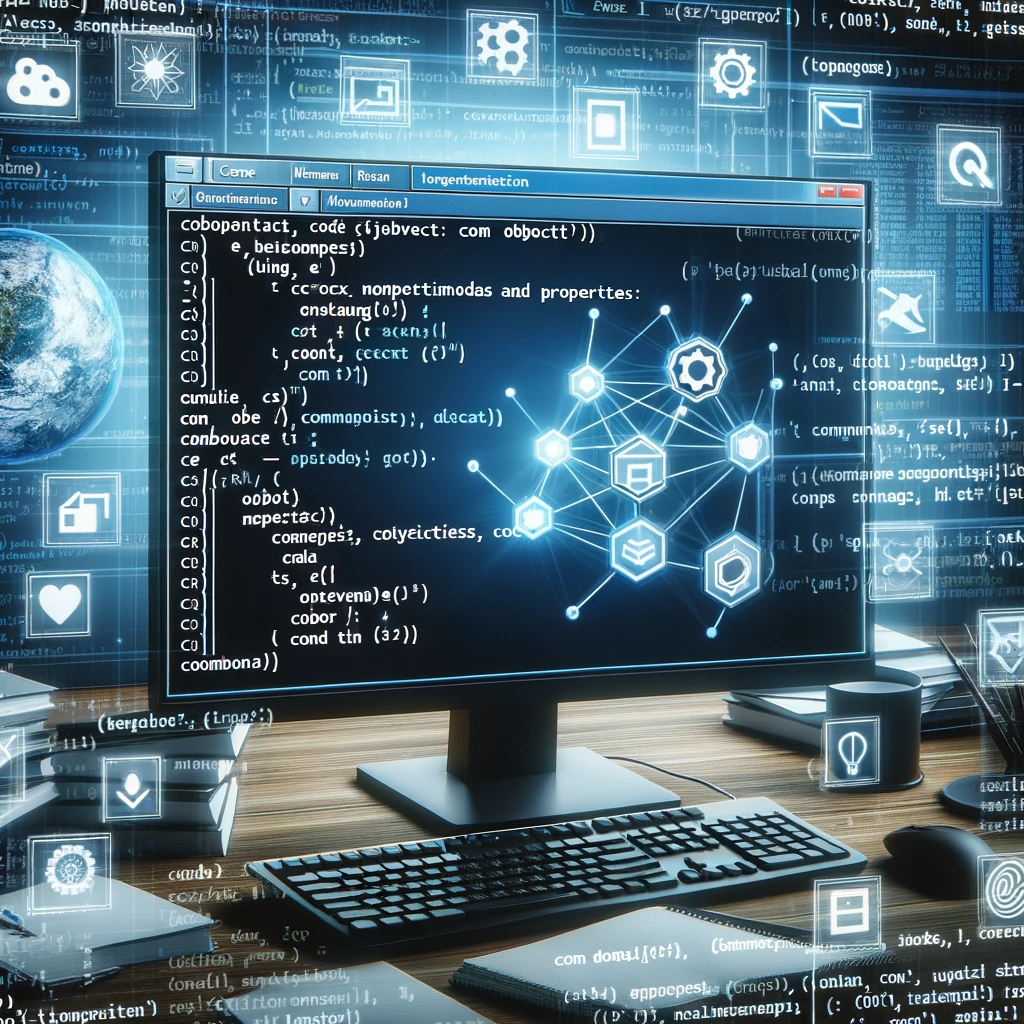
Fundamental Programming Concepts
1. Variables and Their Types
Variables
Imagine a city's mailboxes. Each mailbox (variable) can hold letters (values), but only of a certain size or type (data type). Some are for standard letters, others for parcels, and so on. Just as you can't fit a large package into a small mailbox, you can't store a text string in an integer variable.
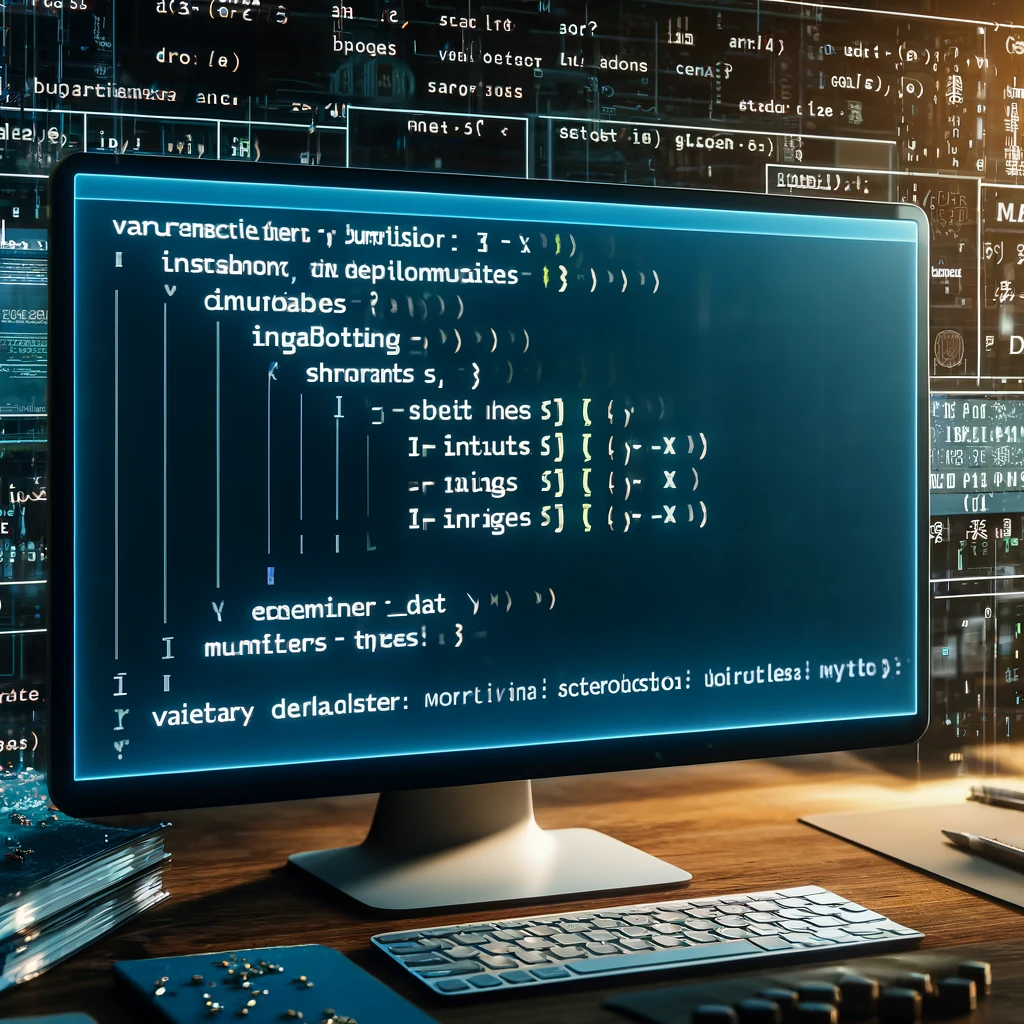
2. Conditionals and Boolean Operations
Conditionals
Think of traffic lights at an intersection. They control the flow based on conditions: if the light is green (true), cars go; if it's red (false), they stop. Conditionals in programming work similarly, directing the flow of execution based on true or false conditions.
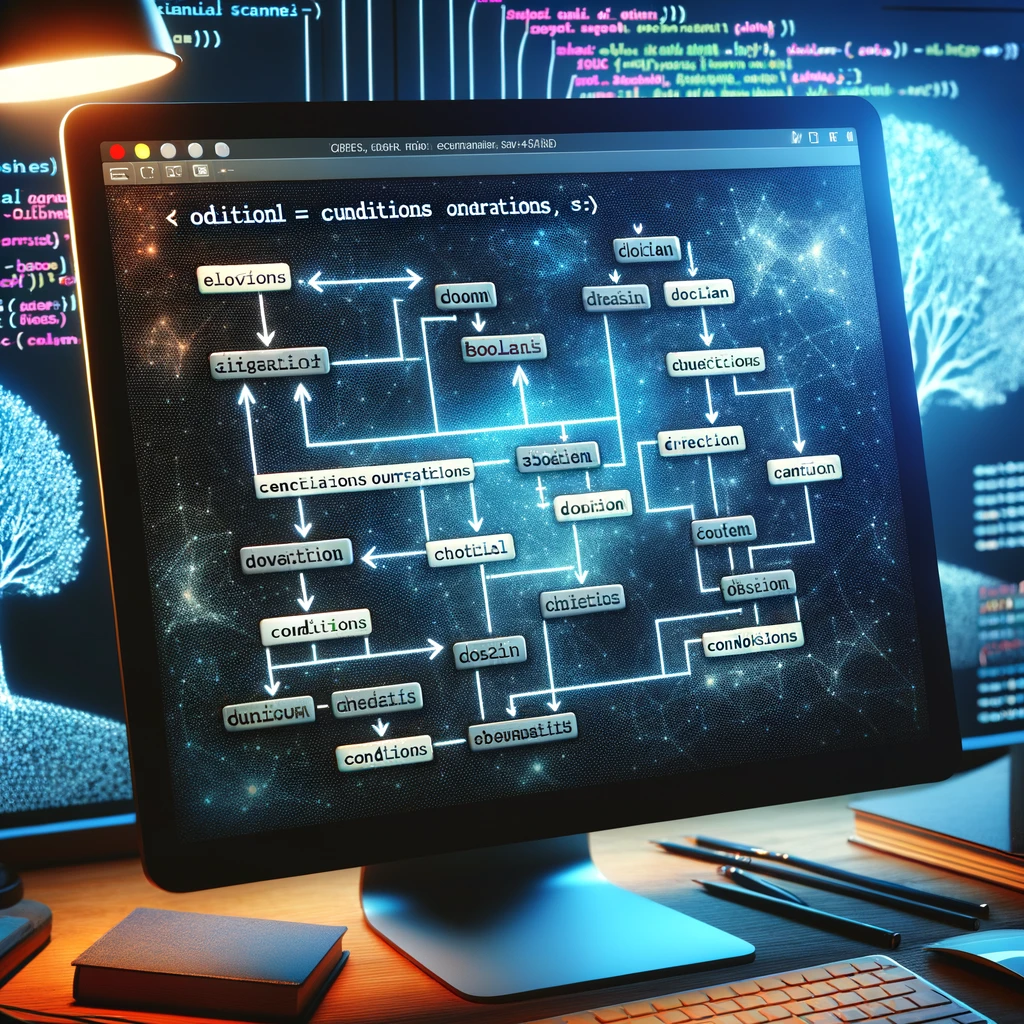
3. Loops and Program Flow
Loops
Consider a roundabout in our metaphorical city. Vehicles (data) enter and exit based on certain conditions, circling (looping) until it's their turn to exit. Similarly, loops in programming keep running a block of code until a condition is met.
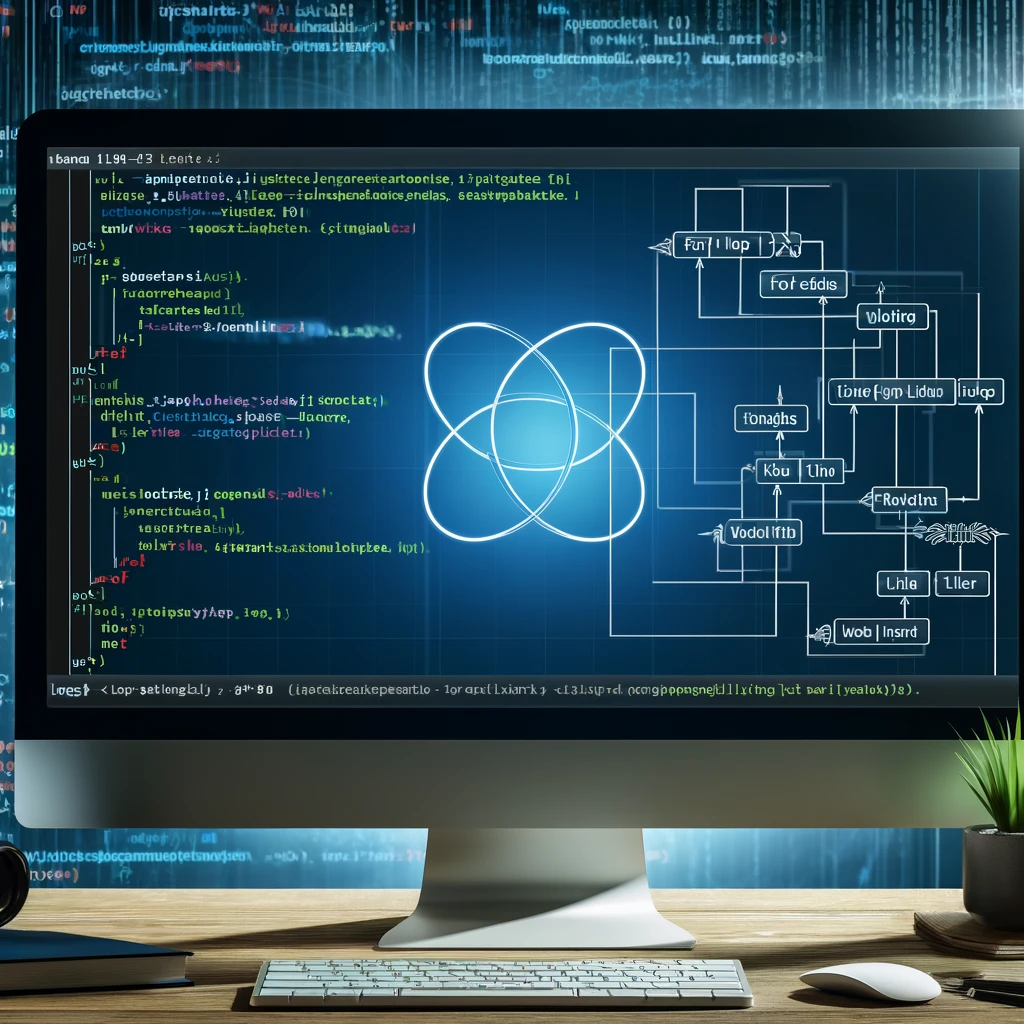
4. Arrays and Lists
Arrays and Lists
Imagine a parking lot. Each parking space (element) is numbered and can hold one vehicle (value). This is akin to arrays and lists, where data is stored in an ordered collection, accessible by an index or key.
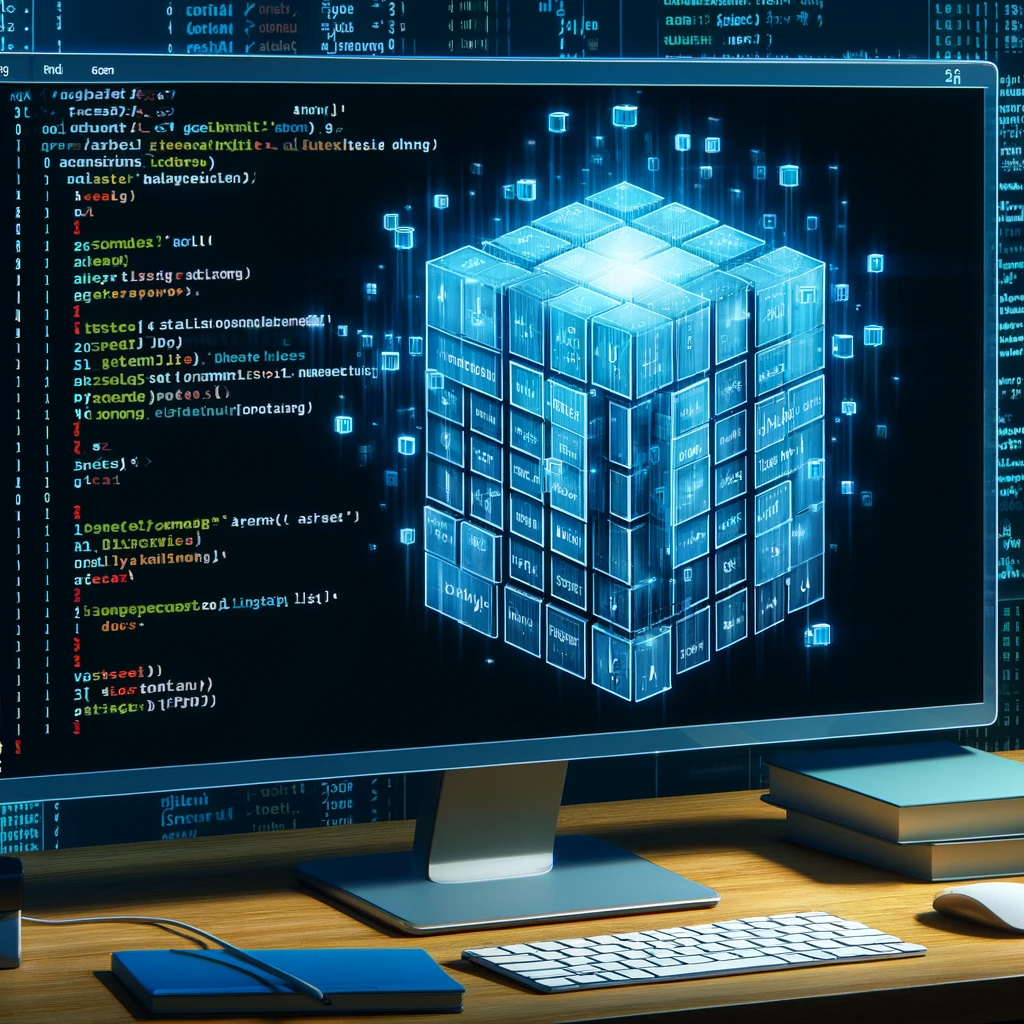
5. Functions and Methods
Functions and Methods
Think of a vending machine. You select a button (call a function) to get a specific snack (output) based on your selection (input). Functions in programming are similar; they perform specific tasks and return results based on the given inputs.
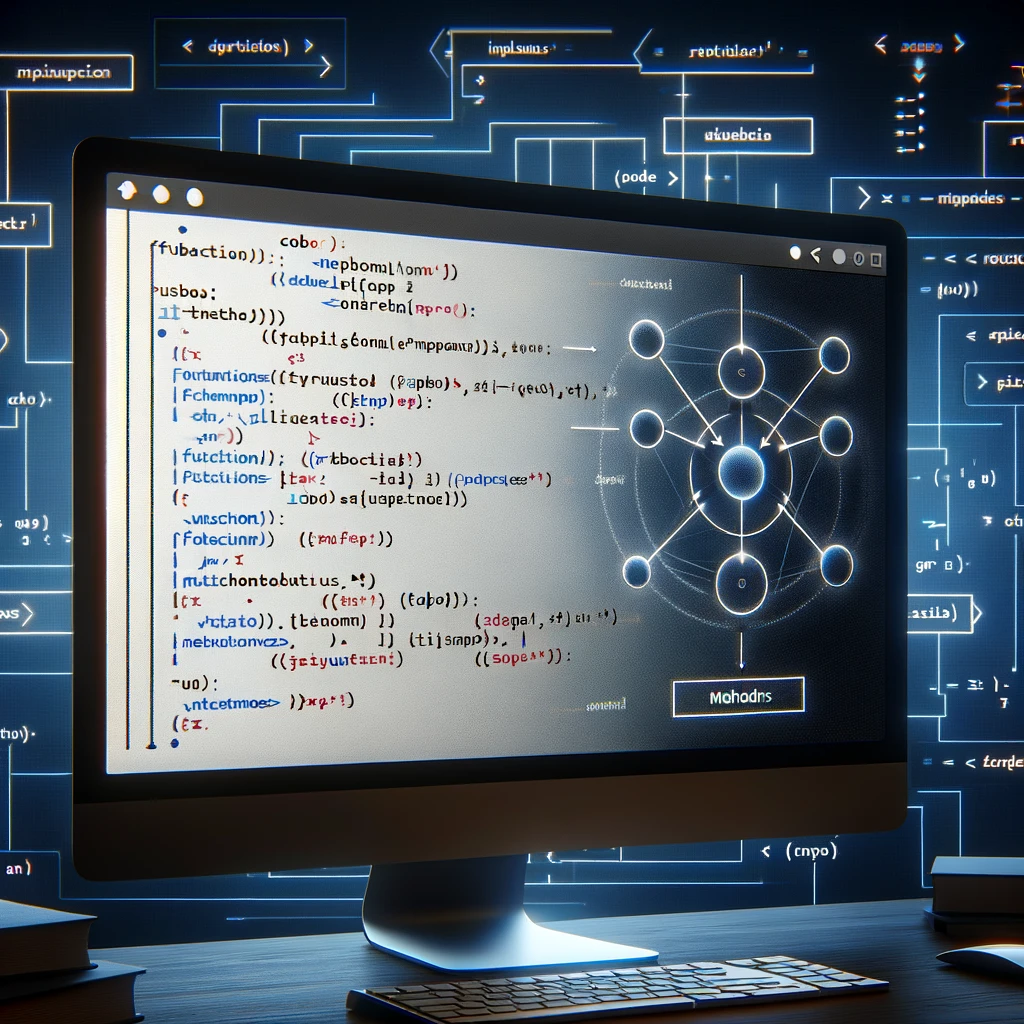
6. File I/O
File I/O
Consider a library. You can borrow (read) books, return them (write), and find them in specific sections (file paths). File Input/Output in programming involves reading from and writing to files, organizing data like books in a library.
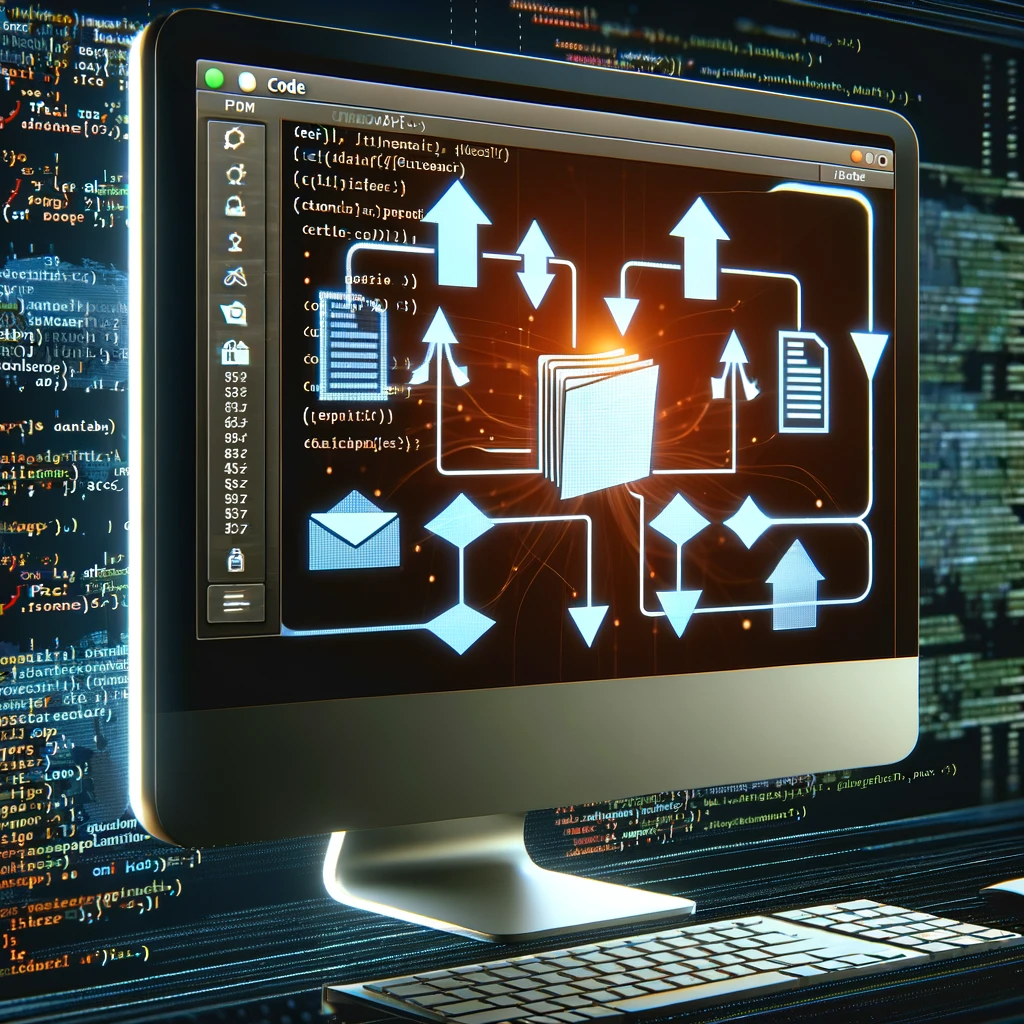
7. Data Structures and Algorithms (DSA)
Data Structures and Algorithms (DSA)
Imagine the city's transportation system, with various vehicles (data structures) like buses, trains, and bikes designed for specific routes (algorithms). Just as choosing the right vehicle and route can get you to your destination more efficiently, selecting the appropriate data structure and algorithm optimizes problem-solving in programming.
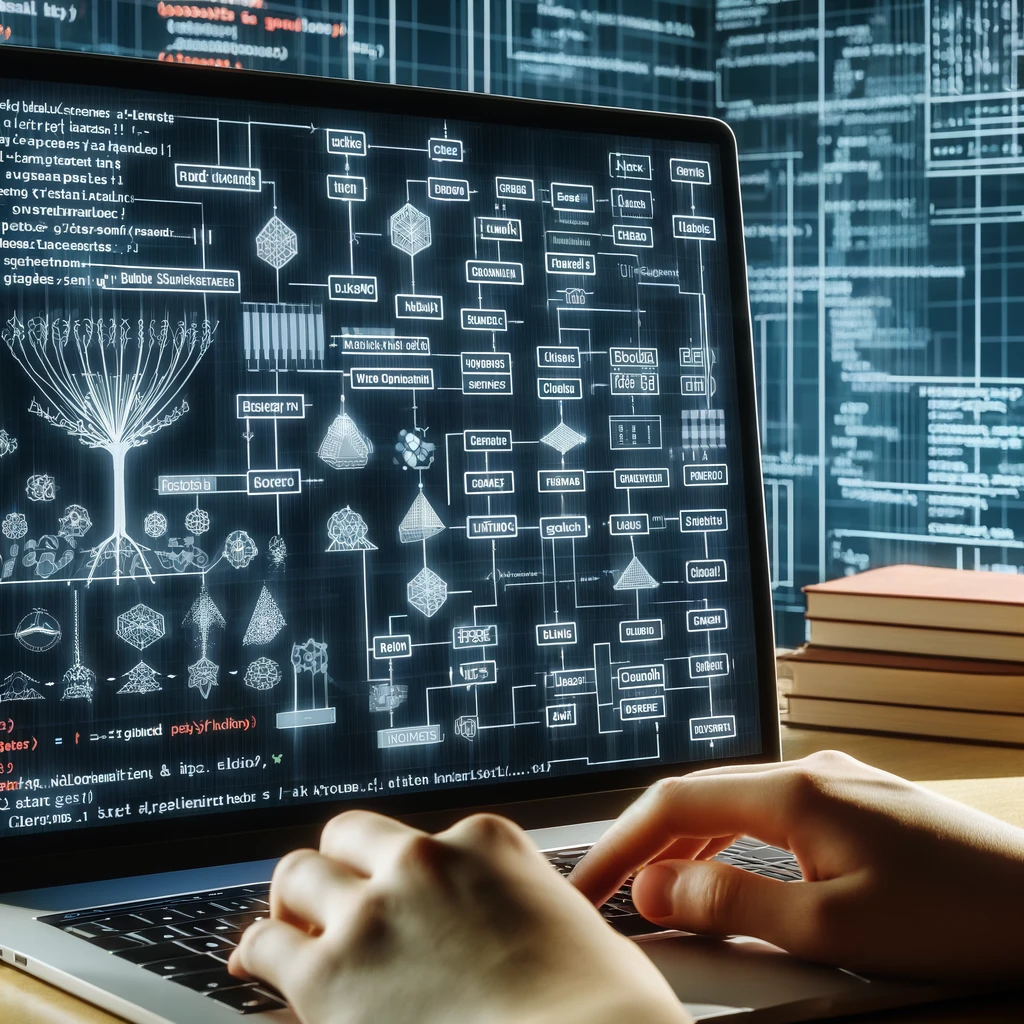
8. Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP)
Consider a city filled with buildings. Each building (object) has a blueprint (class) that defines its structure and functions. Buildings can inherit features from other buildings (inheritance) and have unique characteristics (encapsulation). This mirrors how OOP uses objects and classes to model real-world entities and their interactions.
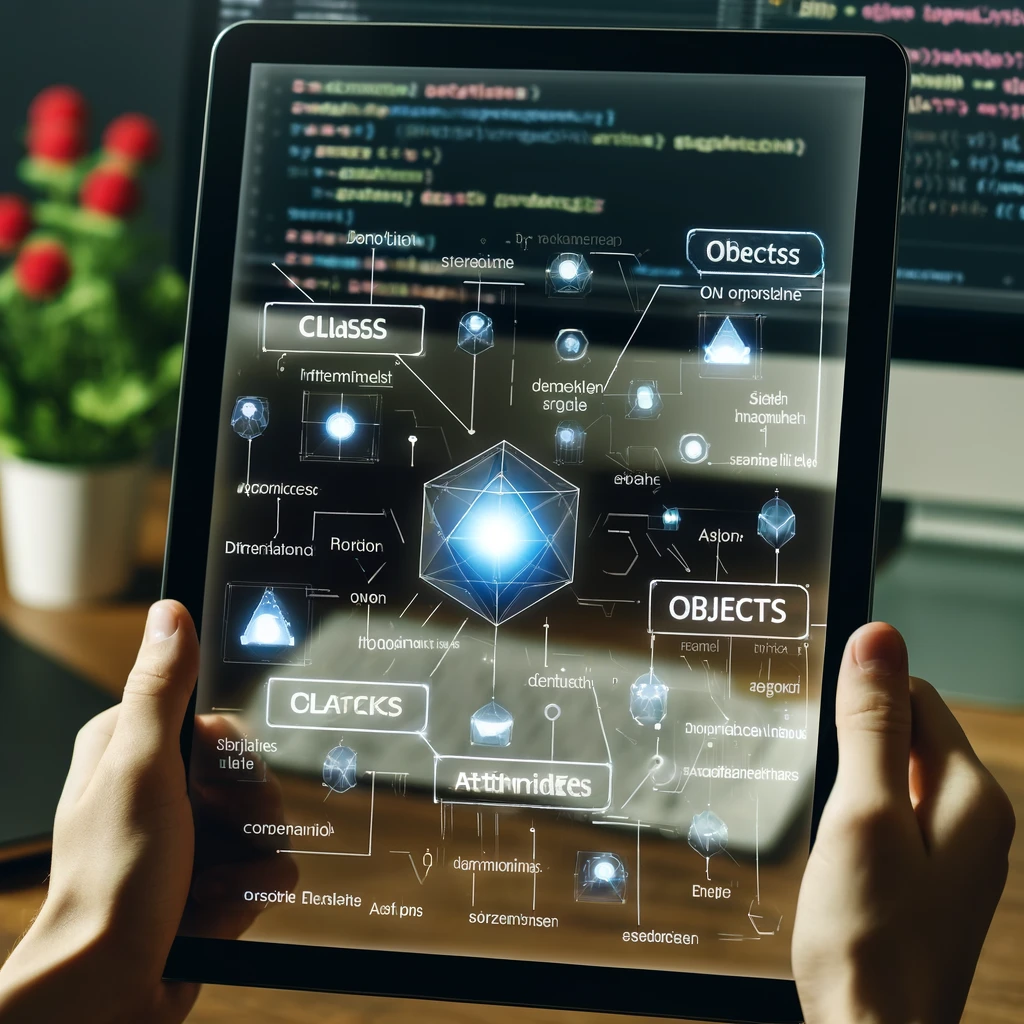
9. Troubleshooting and Debugging
Troubleshooting and Debugging
Imagine a detective solving a mystery in the city. They gather clues (logs), interview witnesses (debugging tools), and piece together what happened to solve the case (fix the bug). Debugging in programming follows a similar investigative process to identify and resolve issues.
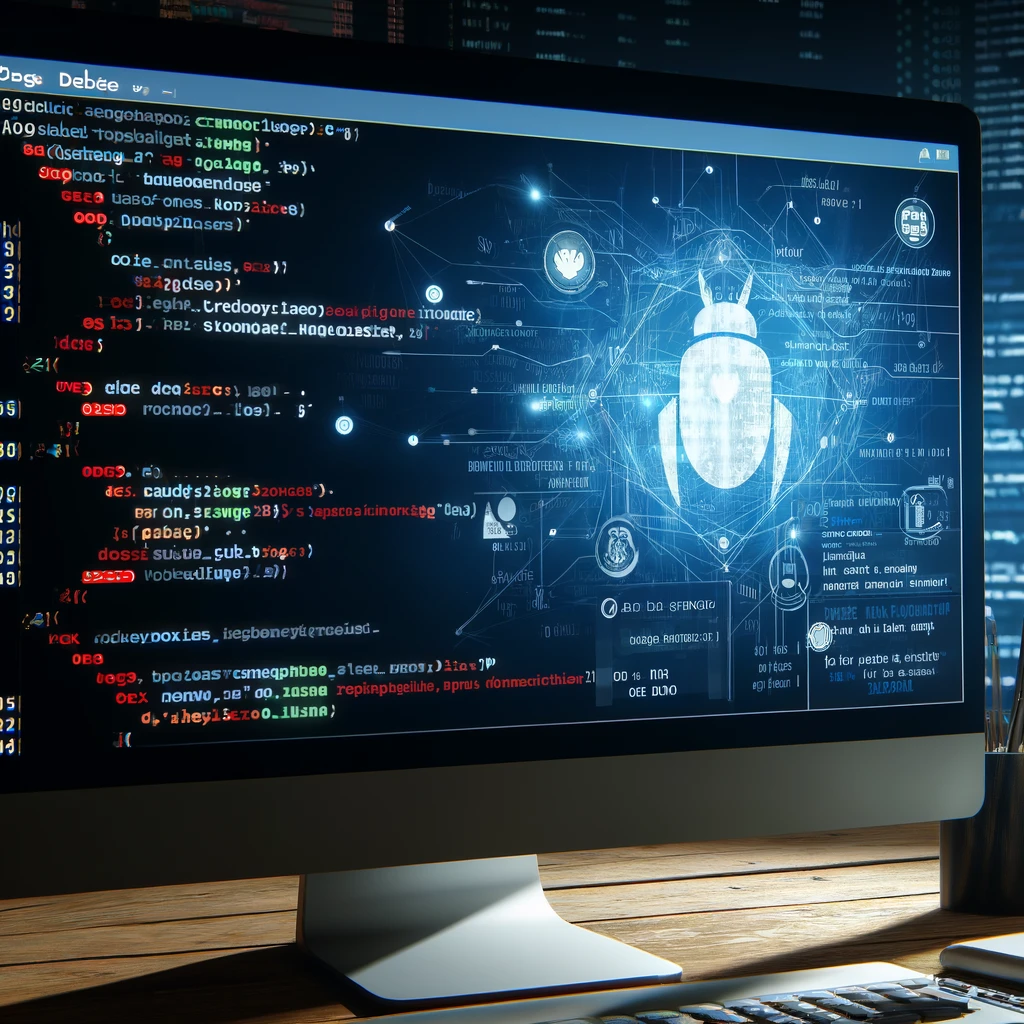
10. Modularity and Abstraction
Modularity and Abstraction
Think of a city's modular homes. Each module (function or class) is designed to be independent but can be combined to build a complex structure (program). Abstraction hides the complexity, showing only the essential features, much like viewing a model home without seeing the wiring and plumbing inside.
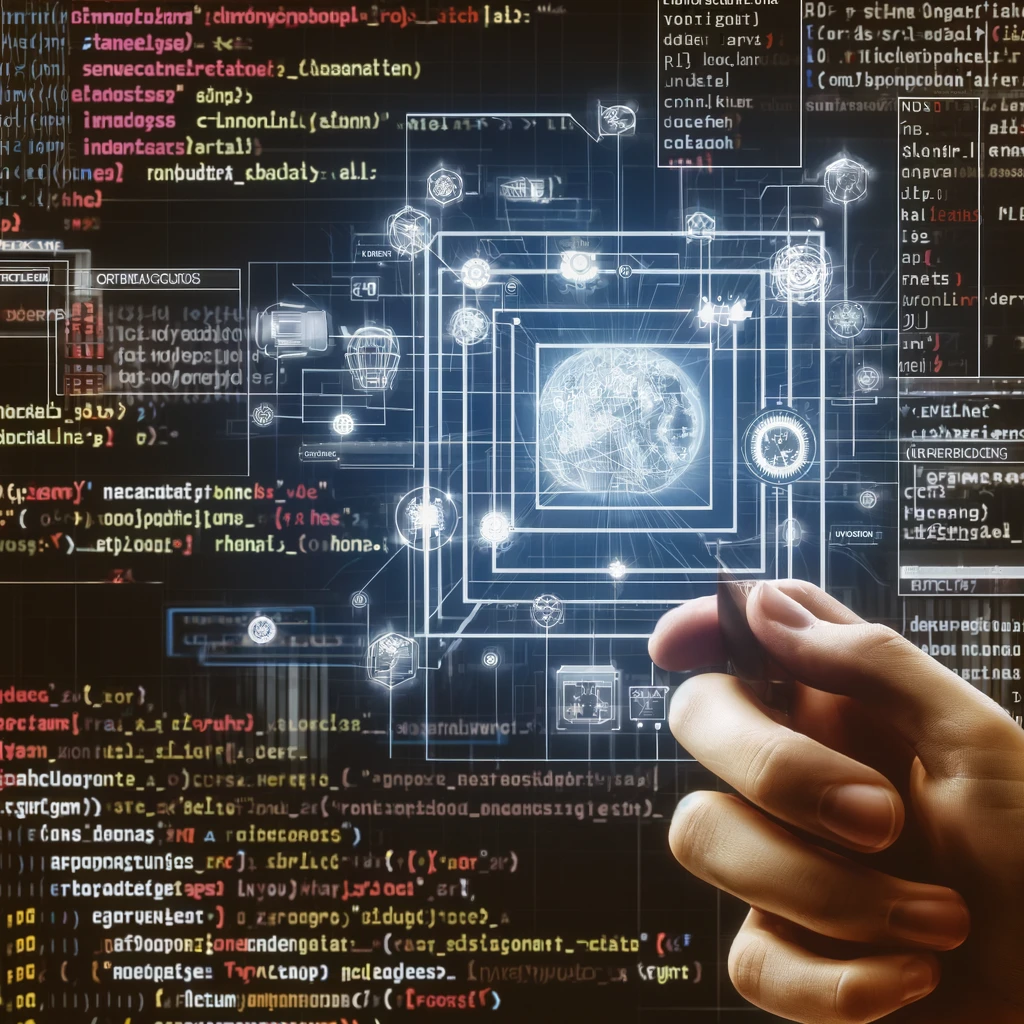
11. Problem Solving and Decomposition
Problem Solving and Decomposition
Consider a city planning committee facing the challenge of urban expansion. They break down the problem into smaller, manageable tasks (decomposition), such as zoning, infrastructure, and public services, to develop a comprehensive plan (solution). Similarly, programmers decompose complex problems into simpler parts to find effective solutions.

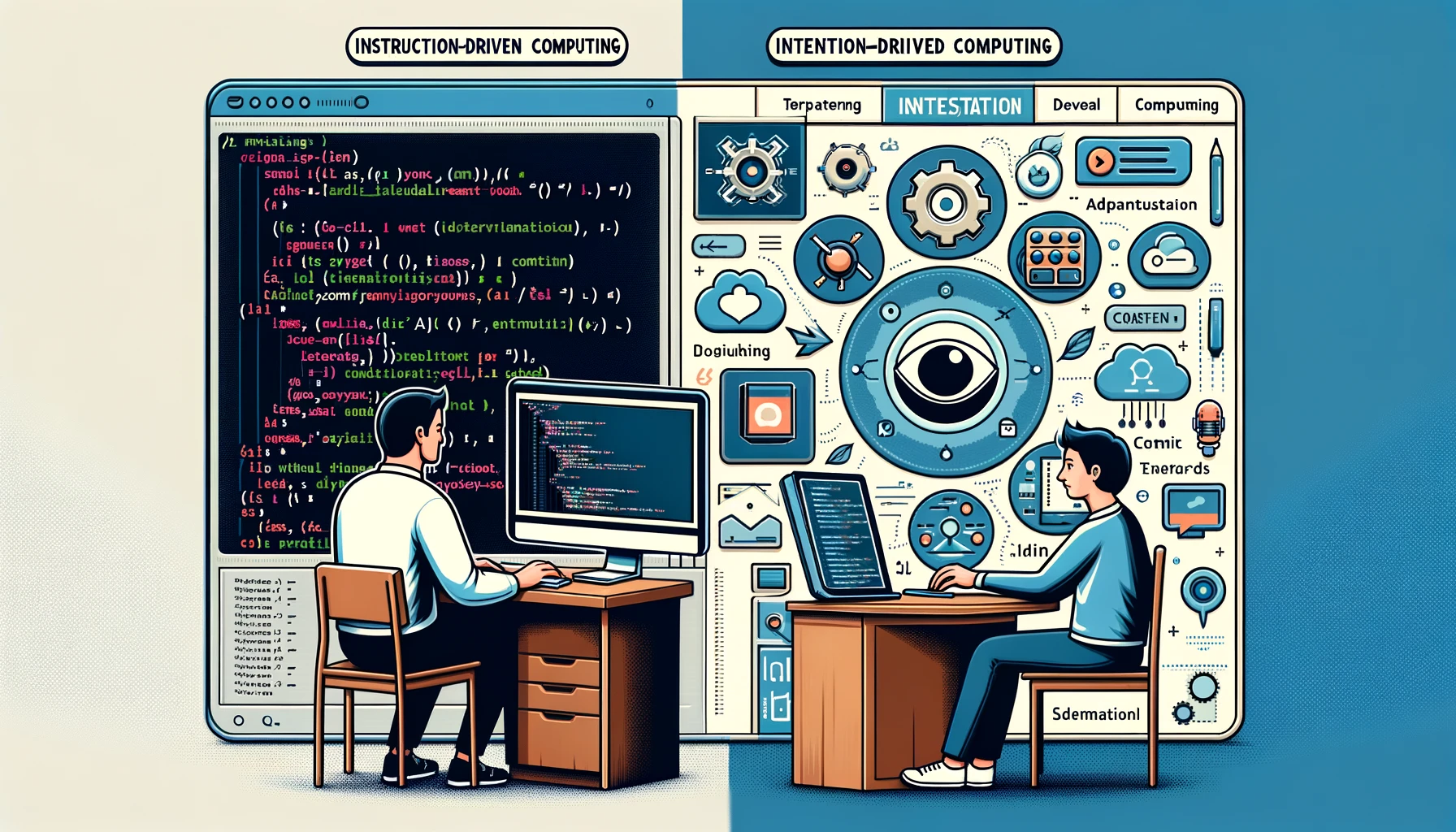
Instruction-driven computing and intention-driven computing represent two different paradigms in how computational tasks are approached and executed. Here’s a detailed comparison:
Instruction-Driven Computing
Definition:
- Instruction-driven computing refers to the traditional method where specific instructions are given to the computer to perform tasks. Each step of the process is explicitly defined by the programmer.
Characteristics:
- Explicit Commands: The user or programmer provides detailed, step-by-step instructions.
- Procedural Approach: Follows a procedural or imperative programming model.
- Predictability: The outcomes are predictable as they strictly follow the given instructions.
- Control: High level of control over how tasks are executed.
- Examples: Traditional programming languages like C, Java, and assembly languages.
Advantages:
- Precision: High precision in task execution.
- Efficiency: Can be optimized for performance.
- Debugging: Easier to trace and debug due to clear instruction flow.
Disadvantages:
- Complexity: Can become complex and difficult to manage for large systems.
- Flexibility: Less flexible in handling unexpected situations or changes in requirements.
Intention-Driven Computing
Definition:
- Intention-driven computing focuses on understanding the user’s intentions and achieving the desired outcomes without requiring explicit step-by-step instructions. It often involves higher-level abstractions and autonomous decision-making.
Characteristics:
- High-Level Goals: Users specify what they want to achieve rather than how to achieve it.
- Declarative Approach: Often follows a declarative programming model where the focus is on the outcome rather than the process.
- Adaptability: Systems are designed to adapt and make decisions based on the context and user intentions.
- Examples: AI-driven systems, natural language processing interfaces, and some aspects of functional programming.
Advantages:
- User-Friendly: Easier for non-programmers to specify what they want.
- Adaptability: Can handle a wide range of scenarios and adapt to changes.
- Efficiency: Reduces the need for detailed programming, allowing faster development.
Disadvantages:
- Ambiguity: Interpreting user intentions can be challenging and may lead to ambiguity.
- Complexity in Implementation: Requires sophisticated algorithms and models to correctly interpret and act on intentions.
- Less Control: Users have less control over the specific steps of execution, which can be a disadvantage in certain scenarios.
Comparison
Aspect | Instruction-Driven Computing | Intention-Driven Computing |
---|---|---|
Approach | Procedural, step-by-step instructions | Declarative, goal-oriented |
User Input | Detailed instructions | High-level goals |
Control | High control over execution | Less control, more autonomy for the system |
Flexibility | Less flexible, rigid | Highly flexible, adaptive |
Complexity | Can become complex with large systems | Complex to implement, but simpler for users |
Examples | Traditional programming languages (C, Java) | AI systems, natural language interfaces, functional programming |
Predictability | Highly predictable outcomes | Outcomes can vary based on interpretation of intentions |
Adaptability | Low adaptability, follows predefined instructions | High adaptability, can handle diverse scenarios |
Conclusion
Instruction-driven computing is ideal for scenarios where precision, control, and predictability are paramount, while intention-driven computing excels in environments that require flexibility, adaptability, and ease of use for end-users. The choice between the two paradigms depends on the specific requirements and context of the task at hand.